QrSnap Chrome extension : smooth transition between desktop and mobile browsers
Posted by Unknown
I have been using an Android device for a couple of weeks, essentially reading RSS feeds and playing Angry Bird :)
My new Chrome extension makes RTE on Google+ a bit easier
Posted by Unknown
If you, hacker, wish to grab the code and bring this extension back to life, I will be delighted to share.

2. Go to your Google+ page,
Looking for a simpler way to transpose a HTML table
Posted by Unknown
An example might be clearer. Lets take this input, a matrix we call A :
A | B |
a | b |
c | d |
A | a | c |
B | b | d |
If this image did not bring you to the point, you can see the operation as the combination of these two :
1. Flipping the matrix horizontally
2. Rotating it counterclockwise by 90°
With that said, lets come back to the point of this post : transposing a HTML Table. But first, why would we need to transpose a table ?
Back to HTML basics
If you are familiar with HTML, you may know (otherwise you are not :) ) that you've got one way to build tables (TABLE tag) : first build rows (TR tag) which you populate with cells (TD tag).
Here is an example of how, in HTML you would create a table with 2 rows, each of which having 2 cells :
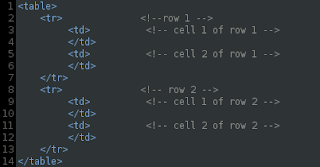
When displaying data fetched from a database, for instance, this paradigm somehow forces you to do it in a certain way.
Since you can't come back to a given line of the code when rendering a html page, you have to be prepared to output top rows first and the bottom ones, after.
For Table 1 above, you would display cell A, then cell B, start another row, display cell a and then cell b and start another row, display cell c and then cell d.
As you can expect, this assume you have the data in this order : [A, B],[a, b], [c, d].
But what if you don't ? I mean you have them in a different order : [A, a, c][B, b, d] for instance, because this is how you are getting the data.
This question can rise from a desgin issue because the visual impact is not the same whether you display a table horizontally or vertically. Take spreadsheets : you can dock a table's header at the top of the page in order to have access to it when scrolling, for example.
How can we answer to this issue ?
Well, you can modify the way you get the data, and folks out there, from the PHP community know how tedious it is to turn a "horizontal" table (where array keys can represent the headers) into a "vertical" one (here, you lose the benefit of having keys, what a shame !)
How jQuery can help ?
Let's assume you decided not to radically change your business objects and made the choice of handling this operation in the client-side.
With what we learned about Matrix Transposition from out basis algebra courses, we will take the problem from HTML the Maths, do the transposition, and come back to HTML ... dead simple !
Here is the process :
Step 1. We will take all cells from the input table and store them into a javascript array (our matrix) , which we call aTd
Step 2. Then we transpose aTd, the resulting array is called aTd_tr.
Step 3. With this new transposed javascript array, we create a new HTML table
First, lets take a look at how we transpose, my goal here was to break the records with the most minimalist function ever (hope i did it :) )
// given a matrix M, computes T, the transpose of M defined as T(i, j) = M(j, i) function transpose(M) { var T = []; for(var i in M) for (var j in M[i]) (T[j] = T[j] || [])[i] = M[i][j]; return T; }
And here is how we use this function to achieve Step 2 and 3 :
$(function(){ // a temporary 2 dimensional array to hold all cells from the original table var aTd = []; var i=0; // loop through all rows : $('#myTable tr').each(function(){ aTd[i] = []; j= 0; // loop through all cell of each row ... $('td', $(this)).each(function(){ // ... and save them : aTd[i][j] = $(this).clone(); j++; }) i++; }) // transpose the array (see 'transpose' function above) aTd_tr = transpose(aTd); // create a new empty table var newTable = $('<_table>'); // remove spaces and underscores // populate it with cells from the transposed matrix : $.each(aTd_tr, function(index1){ var tr = $('<_tr>'); // remove underscores newTable.append(tr); $.each(aTd_tr[index1], function(index2, value2){ tr.append(value2); }) }); $('body').append(newTable); })
We are done !
Taking advice from my buddy Cedric, I would have showcased this technique but I guess you know what the result would look like : see Table 1 and Table 2 higher above :)
Hope you enjoyed this post. Feel free to feed me back at assane101 at gmail
jql : Javascript Query Language
Posted by Unknown
Last night I jumped out the bed with a blurry idea of what an implementation of a SQL-like query would look like.
I booted my computer and had a look at jQuery's code to see how it was actually querying the DOM.
For those who don't already know about it, SQL stands for Structured Query Language and is designed to abstract operations required to retrieve data stored into databases.
It has 3 main operations you can use to run queries : FROM, SELECT and WHERE, that are used in this way : "FROM some_table SELECT some_column WHERE another_column=some_value".
some_table could be a table where you store, for instance, information about users of you website, lets call it users_table. It will be holding data like user's email, login, password, username, ... for example.
Then, when you later need to pull a set of information or all the data related to a given user (assuming you know his email, let's say foo@bar.com ), you will query you database using SQL like this : "FROM users_table SELECT login, password WHERE email='foo@bar.com'".
This will retrieve the login and password from the list of entries in users_table for the specific user whose email is 'foo@bar.com'.
This is a rough presentation of what SQL is about, you may make a search on Google to find more information.
Coming back to jQuery, I was wondering if this approach of manipulating data could be applied to DOM elements (in a HTML webpage) in some way like this :
var myQuery = $.select("a").from("body").where("href^='http://'")
.. and have it pull all links descending from the body element and which href attribute starts whith "htt://".
myQuery would then be jQuery object you can then be manipulated in the usual way. Adding a CSS class to all of them would be done this way :
myQuery.addClass('selected');
For this job to be possible, I extended jQuery, adding from, select and where methods.
I also added a get method by which you tell "I am done specifying my query, now you can run it !", which is equivalent to hitting return key in a command line SQL program or calling mysql_query in PHP.
The select method takes a css selector string as unique argument, and should be called first. It stores the selector for subsequent use by get.
Same as select, the from method also stores the given selector.
This implementation of JQL expects one selector for select and from, and as much calls to where as you want, enabling you to filter content through different attributes like width, height, ...
jQuery.extend({
select : function(selector){
this.jql_select = selector || "*";
this.jsql_from = "body";
this.jsql_where = "";
return this;
},
from : function(selector){
this.jsql_from = selector || "body";
return this;
},
where : function(condition){
switch (typeof condition)
{
case 'string':
this.jsql_where = (this.jsql_where||"")+"[" + condition + "]";
break;
case 'object':
var str_filter = "";
jQuery.each(condition, function(key, value){
if(jQuery.inArray(value[0], ["=", "!=", "^=", "$=", "*="]) == -1)
{
alert("unknown where compare operator : "+value[0]);
}
else if(jQuery.inArray(key, ["width", "height"]) == -1)
{
alert("unknown where attribute : "+value[1]);
}else{
str_filter += key.toString()+value[0]+value[1];
}
});
this.jsql_where = (this.jsql_where||"")+"[" + str_filter + "]";
break;
}
return this;
},
get : function(){
return jQuery(this.jql_select, this.jsql_from).filter(this.jsql_where);
}
})
Here is an example of how you can call these functions :
var elements =
jQuery.select("*")
.from("body")
.where("width=200") // equivalent to .where({width : ["=", 200]})
.get();
console.log(elements.length+" elements where returned");
console.log(elements.attr("id"));
Of course, you can imagine (and do !) extending with conditions like ">", ">=", "<" and "<=". I think you'll need to loop through the jQuery set after selecting and test each of such conditions. As a conclusion I would like to point out the fact that this is not an improvement to jQuery. It rather tries to showcase how easy it is to implement a SQL-like paradigm in jQuery. I hope you'll enjoy reading this post.
Coming next ..
Next time we will be talking about how to transpose a html table, turning it from horizontal to vertical, and vice versa, with jQuery of course ... keep tuned !
Drag&Drop : the least obvious way
Posted by assaneonline
Today, playing with my Google Chrome browser (which, btw I do so frequently ;) ) I observered that dragging file from my desktop and dropping them onto a HTML file upload input ( ) was actually selecting the file. In other words, this drag-and-drop is equivalent to clicking the file upload button, browsing the local file explorer and choosing the file. Isn't this great ?
Going beyond this simple observation
Once you have done this, you can imagine what's next : handling the file upload from the server, saving the file, displaying it if necessary, ....
Going further beyond
- This works well in Chrome but not in my version of Firefox (3.6.13) which actaully tries to open the file you drop onto the page.
- HTML5 brings an implementation of drag-and-drop you can test here assuming you have the rigth browser (you can see your browser's HTML5 support here : http://html5test.com).
[Gmail hack] Spicing your signature
Posted by assaneonline
Gmail recently added a rich text functionality to its signature editor. Until then, you could only have an insipid text, with no formatting. This was a huge enhancement.
Here is a screenshot of the current editor :
But if you want to style your signature a little bit, say you want to add a complex structure, such as a two column signature, you're unlucky !
The solution I came out with is more or less geeky one and will require you to know some HTML or have a less limited HTML editor.
For both cases you'll need to have Firebug installed. It's a Firefox addon you can get here : http://bit.ly/aZ0pa1.
Next :
1. Navigate to your "Settings" by clicking on the link at the top right corner of your GMail page : you end up in you General settings page.
Scroll down to the signature box.
2. Activate Firebug if it's not already on (you general hit F12 to fire it up).
Then click on the inspector pointer within Firebug to start exploring the content of the Gmail Settings page.
You're now inspecting the code, and Firebug automaticaly update whenever you hover a element of the page with the pointer.
3. Then click on the signature box to inspect it.
4. Go back to the Firebug's frame, make you you select the body element into the iframe wrapping the signature editor. Then right click on the corresponding html element and select "Edit HTML" in the menu.
5. Depending on you being an HTML expert excited about working around a Google limitation or a newbie afraid of all these coding stuffs, you can either edit the HTML content of you signature right into Firebug HTML Editor or copy and paste it from your favourite WYSIWYG Editor.
Make sure you style it properly and don't have your CSS tied to a external stylesheet.
That's it, for today, hope you'll come up with a GMail signature, much nicer than mine below :
Here is the HTML code I used :
[CSS Trick] Disable list-style for an 'one item list'
Posted by assaneonline
This is an issue I run into during my internship (which, by the way is still going on quite well :) ).
Purged from the details of how did the problem rise, here is what it is :
I have a set or data I wanna display using a HTML list (ol or ul).
This is what it looks like when every thing is OK :
- Data one
- Data two
- Data three
The problem emerge when the set contains one item, because it look a little weird to have the bullet point in a list that contains one item, in other words 'a list that is not a list :s !'.
(You can think this is not a big deal, but the meticulous [or perhaps crazy] guy who I am can't let this go).
This is the solution I came up with (be sure that I Googled the stuff to be sure it hasn't been solved elsewhere), and it is pure CSS :) :
- Single item :(
#theList li:first-child:last-child{
list-style:none;
}