Looking for a simpler way to transpose a HTML table
Posted by Unknown
In Mathematics, transposing a matrix (a two dimensional set of data with columns and rows) is the operation by which you interchange rows and columns, resulting in a new matrix.
An example might be clearer. Lets take this input, a matrix we call A :
Table 1
The transpose of this matrix would then be :
Table 2
If this image did not bring you to the point, you can see the operation as the combination of these two :
1. Flipping the matrix horizontally
2. Rotating it counterclockwise by 90°
With that said, lets come back to the point of this post : transposing a HTML Table. But first, why would we need to transpose a table ?
Back to HTML basics
If you are familiar with HTML, you may know (otherwise you are not :) ) that you've got one way to build tables (TABLE tag) : first build rows (TR tag) which you populate with cells (TD tag).
Here is an example of how, in HTML you would create a table with 2 rows, each of which having 2 cells :
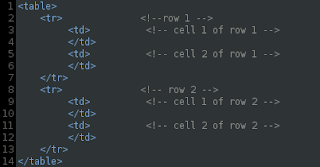
When displaying data fetched from a database, for instance, this paradigm somehow forces you to do it in a certain way.
Since you can't come back to a given line of the code when rendering a html page, you have to be prepared to output top rows first and the bottom ones, after.
For Table 1 above, you would display cell A, then cell B, start another row, display cell a and then cell b and start another row, display cell c and then cell d.
As you can expect, this assume you have the data in this order : [A, B],[a, b], [c, d].
But what if you don't ? I mean you have them in a different order : [A, a, c][B, b, d] for instance, because this is how you are getting the data.
This question can rise from a desgin issue because the visual impact is not the same whether you display a table horizontally or vertically. Take spreadsheets : you can dock a table's header at the top of the page in order to have access to it when scrolling, for example.
How can we answer to this issue ?
Well, you can modify the way you get the data, and folks out there, from the PHP community know how tedious it is to turn a "horizontal" table (where array keys can represent the headers) into a "vertical" one (here, you lose the benefit of having keys, what a shame !)
How jQuery can help ?
Let's assume you decided not to radically change your business objects and made the choice of handling this operation in the client-side.
With what we learned about Matrix Transposition from out basis algebra courses, we will take the problem from HTML the Maths, do the transposition, and come back to HTML ... dead simple !
Here is the process :
Step 1. We will take all cells from the input table and store them into a javascript array (our matrix) , which we call aTd
Step 2. Then we transpose aTd, the resulting array is called aTd_tr.
Step 3. With this new transposed javascript array, we create a new HTML table
First, lets take a look at how we transpose, my goal here was to break the records with the most minimalist function ever (hope i did it :) )
And here is how we use this function to achieve Step 2 and 3 :
We are done !
Taking advice from my buddy Cedric, I would have showcased this technique but I guess you know what the result would look like : see Table 1 and Table 2 higher above :)
Hope you enjoyed this post. Feel free to feed me back at assane101 at gmail
An example might be clearer. Lets take this input, a matrix we call A :
A | B |
a | b |
c | d |
A | a | c |
B | b | d |
If this image did not bring you to the point, you can see the operation as the combination of these two :
1. Flipping the matrix horizontally
2. Rotating it counterclockwise by 90°
With that said, lets come back to the point of this post : transposing a HTML Table. But first, why would we need to transpose a table ?
Back to HTML basics
If you are familiar with HTML, you may know (otherwise you are not :) ) that you've got one way to build tables (TABLE tag) : first build rows (TR tag) which you populate with cells (TD tag).
Here is an example of how, in HTML you would create a table with 2 rows, each of which having 2 cells :
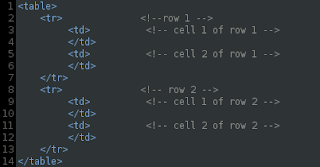
When displaying data fetched from a database, for instance, this paradigm somehow forces you to do it in a certain way.
Since you can't come back to a given line of the code when rendering a html page, you have to be prepared to output top rows first and the bottom ones, after.
For Table 1 above, you would display cell A, then cell B, start another row, display cell a and then cell b and start another row, display cell c and then cell d.
As you can expect, this assume you have the data in this order : [A, B],[a, b], [c, d].
But what if you don't ? I mean you have them in a different order : [A, a, c][B, b, d] for instance, because this is how you are getting the data.
This question can rise from a desgin issue because the visual impact is not the same whether you display a table horizontally or vertically. Take spreadsheets : you can dock a table's header at the top of the page in order to have access to it when scrolling, for example.
How can we answer to this issue ?
Well, you can modify the way you get the data, and folks out there, from the PHP community know how tedious it is to turn a "horizontal" table (where array keys can represent the headers) into a "vertical" one (here, you lose the benefit of having keys, what a shame !)
How jQuery can help ?
Let's assume you decided not to radically change your business objects and made the choice of handling this operation in the client-side.
With what we learned about Matrix Transposition from out basis algebra courses, we will take the problem from HTML the Maths, do the transposition, and come back to HTML ... dead simple !
Here is the process :
Step 1. We will take all cells from the input table and store them into a javascript array (our matrix) , which we call aTd
Step 2. Then we transpose aTd, the resulting array is called aTd_tr.
Step 3. With this new transposed javascript array, we create a new HTML table
First, lets take a look at how we transpose, my goal here was to break the records with the most minimalist function ever (hope i did it :) )
// given a matrix M, computes T, the transpose of M defined as T(i, j) = M(j, i) function transpose(M) { var T = []; for(var i in M) for (var j in M[i]) (T[j] = T[j] || [])[i] = M[i][j]; return T; }
And here is how we use this function to achieve Step 2 and 3 :
$(function(){ // a temporary 2 dimensional array to hold all cells from the original table var aTd = []; var i=0; // loop through all rows : $('#myTable tr').each(function(){ aTd[i] = []; j= 0; // loop through all cell of each row ... $('td', $(this)).each(function(){ // ... and save them : aTd[i][j] = $(this).clone(); j++; }) i++; }) // transpose the array (see 'transpose' function above) aTd_tr = transpose(aTd); // create a new empty table var newTable = $('<_table>'); // remove spaces and underscores // populate it with cells from the transposed matrix : $.each(aTd_tr, function(index1){ var tr = $('<_tr>'); // remove underscores newTable.append(tr); $.each(aTd_tr[index1], function(index2, value2){ tr.append(value2); }) }); $('body').append(newTable); })
We are done !
Taking advice from my buddy Cedric, I would have showcased this technique but I guess you know what the result would look like : see Table 1 and Table 2 higher above :)
Hope you enjoyed this post. Feel free to feed me back at assane101 at gmail
Inscription à :
Publier les commentaires (Atom)